Automation Object Model in UFT is a way to automate UFT itself using the COM interface. The automation object model is a set of objects, methods, and properties that can be used to control the configuration settings and execute the scripts using the UFT interface. You can accomplish various things using UFT Automation Object Model. The list is very long, but here are a few examples.
Load the required add-ins for a Test using Automation Object Model in UFT
Example: The following example shows loading the required add-in for the Test and launch the UFT with the visible mode and open an existing Test and run it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
Set qtApp= CreateObject("QuickTest.Application") 'Specify which add-ins to load arrOfAddins = Array("Web,Visual Basic,WPF") 'Loading three add-ins 'The addins listed in the array will be loaded when UFT starts. qtApp.SetActiveAddins(arrTestAddins) qtApp.Launch 'Start UFT qtApp.Visible = True 'Makes the UFT visible qtApp.Open "E:\UFT_WorkSpace\TestScripts\LoginToMyFlight" 'Open the test qtApp.Test.Run 'Run the UFT test qtApp.Test.Close 'Close the UFT test qtApp.Quit 'Close UFT Set qtApp= Nothing ' Release the UFT Application object |
Run UFT in Minimized Mode Using Automation Object Model in UFT
Associate Required Object Repositories using Automation Object Model in UFT
qtApp.Test.Actions(“Action Name”).ObjectRepositories.Add “<Repository Path>”
1 |
qtApp.Test.Actions("abcd").ObjectRepositories.Add "D:\Repository.tsr" |
Associate Required Function Libraries Using Automation Object Model in UFT
To associate a function library with a Test using UFT Automation Object Model we can use the following code
Syntax
qtApp.Test.Settings.Resources.Libraries.Add(“<Func Lib Path>”)
1 |
qtApp.Test.Settings.Resources.Libraries.Add("E:\UFT_WorkSpace\FunctionLib\CommonFunctions.txt") |
Set the Common Object Sync Time Out Using Automation Object Model in UFT
Set Start and End Iteration of DataTable Using Automation Object Model in UFT
1 |
qtApp.Test.Settings.Run.IterationMode = "rngAll" |
The syntax for running a Test for the specified range of rows.
1 2 3 4 5 |
qtApp.Test.Settings.Run.IterationMode = "rngIterations" qtApp.Test.Settings.Run.StartIteration = 1 ' From first row qtApp.Test.Settings.Run.EndIteration = 5 ' fifth row |
Enable/Disable Smart Identification Using Automation Object Model in UFT
1 |
qtApp.Test.Settings.Run.DisableSmartIdentification = True 'To disable set False |
Set On Error Settings Using Automation Object Model in UFT
1 |
qtApp.Test.Settings.Run.OnError = "Dialog" 'Display error message in the dialogbox |
Or
1 |
qtApp.Test.Settings.Run.OnError = "NextStep" 'Instruct UFT to perform next step when error occurs |
Set DataTable Path Using Automation Object Model in UFT
1 |
qtApp.Test.Settings.Resources.DataTablePath = "" |
Add Recovery Scenario Using Automation Object Model in UFT
We can easily add a recovery scenario at runtime using the Automation Object Model (AOM). Use the following syntax
qtApp.Test.Settings.Recovery.SetActivationMode “OnError“
qtApp.Test.Settings.Recovery.Add “Path of Recovery scenario file“, “Recovery Scenario Name“, 1
qtApp.Test.Settings.Recovery.Item(1).Enabled = True
1 2 3 4 5 |
qtApp.Test.Settings.Recovery.SetActivationMode "OnError" 'Create qtApp object first qtApp.Test.Settings.Recovery.Add "E:\UFT_WorkSpace\HandleAutoCompletePopup.qrs", "HandleAutoCompletePopup", 1 qtApp.Test.Settings.Recovery.Item(1).Enabled = True |
Add Multiple Recovery Scenarios Using Automation Object Model in UFT
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
Set qtApp = CreateObject("QuickTest.Application") Set qtRecovery = qtApp.Test.Settings.Recovery ' Returns the Recovery object qtApp.Test.Settings.Recovery.SetActivationMode "OnError" qtApp.Test.Settings.Recovery.Add "E:\UFT_WorkSpace\TestRecovery.qrs", "HandleAutoCompletePopup", 1 qtApp.Test.Settings.Recovery.Add "E:\UFT_WorkSpace\TestRecovery.qrs", "AlertPopup", 2 qtApp.Test.Settings.Recovery.Add "E:\UFT_WorkSpace\TestRecovery.qrs", "RuntimeError", 3 'Enable all Recovery Scenarios For i = 1 To qtRecovery.Count qtRecovery.Item(i).Enabled = True ' Enable each Recovery Scenario one by one Next |
Capture Image in the Test Result for Failed steps Using Automation Object Model
qtApp.Options.Run.ImageCaptureForTestResults = “OnError”
Finding All Actions in a Test and Run the Specified one Using AOM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
Set qtApp = CreateObject("QuickTest.Application") qtApp.Launch qtApp.Visible = True qtApp.Options.Run.ImageCaptureForTestResults = "OnError" qtApp.Options.Run.RunMode = "Fast" qtApp.Options.Run.ViewResults = False qtApp.Open "E:\UFT_WorkSpace\TestScripts\MultipleActionTest", True iActCounter=qtApp.Test.Actions.Count msgbox "total number of actions is " & iActCounter For iCounter=1 to iActCounter ' Action index start from 1 If INSTR(1,(qtApp.Test.Actions(iCounter).Name),"Action2",1) > 0 Then 'Run Action if Action name is Action2 qtApp.Test.RunAction qtApp.Test.Actions(iCounter).Name End If Next qtApp.Test.Close qtApp.Quit Set qtApp = Nothing |
Running a Test and specify it’s test result location Using AOM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
Set qtApp = CreateObject("QuickTest.Application") qtApp.Launch ' Start UFT qtApp.WindowState = "Maximized" qtApp.Visible = True ' Makes the UFT visible qtApp.Options.Run.ImageCaptureForTestResults = "OnError" qtApp.Options.Run.RunMode = "Fast" qtApp.Options.Run.ViewResults = False 'qtApp.Test.SaveAs "E:\UFT_WorkSpace\TestScripts\MultipleActions" ' Save the test qtApp.Open "E:\UFT_WorkSpace\TestScripts\MultipleActions", True ' Open the test in read-only mode Set qtTest = qtApp.Test Set UFTResultsOpt = CreateObject("QuickTest.RunResultsOptions") ' Create the Run Results Options object UFTResultsOpt.ResultsLocation = "E:\UFT_WorkSpace\TestScripts\MultipleActions\Res1" ' Set the results location qtTest.Run UFTResultsOpt ' Run the test with result option qtApp.Test.Close 'Close the UFT test qtApp.Quit 'Close UFT Set qtTest = Nothing Set qtApp = Nothing ' Release the UFT Application object |
Create User-Defined Environment Variable at Runtime and Save a Test
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
Set qtApp = CreateObject("QuickTest.Application") qtApp.Launch ' Start UFT qtApp.WindowState = "Maximized" qtApp.Visible = True ' Makes the UFT visible qtApp.Open "E:\UFT_WorkSpace\TestScripts\GUITest1", False qtApp.Options.Run.ImageCaptureForTestResults = "OnError" qtApp.Options.Run.RunMode = "Fast" qtApp.Options.Run.ViewResults = False qtApp.Test.Environment.Value("TestMgmtTool") = "HP ALM" qtApp.Test.Environment.Value("AutomationTool") = "UFT" qtApp.Test.SaveAs "E:\UFT_WorkSpace\TestScripts\MultipleActions" ' Save the test qtApp.Test.Close 'Close the UFT test qtApp.Quit 'Close UFT Set qtApp = Nothing ' Release the UFT Application object |
After running the test if you will open UFT and go to Test Setting>Environment and select User-Defined variable type, you will see that the above-mentioned environment variables have been added.
Get Details of all Associated Function Libraries to a Test Using AOM
Suppose you have a GUI Test and want to retrieve the details of all associated function libraries in the Test. If any of the required libraries are not associated then load it at runtime and continue with the Test flow. The following piece of code can be written inside a Test in UFT.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
Set qtApp = CreateObject("QuickTest.Application") Set qtLibraries = qtApp.Test.Settings.Resources.Libraries ' Set the libraries collection object FlagLib=False For i = 1 To qtLibraries.Count ' Add TestLib.txt if it's not associated in Test Settings If qtLibraries.Item(i) = "E:\UFT_WorkSpace\FunctionLib\TestLib.txt" Then FlagLib=True Exit For End If Next If FlagLib = False Then LoadFunctionLibrary "E:\UFT_WorkSpace\FunctionLib\TestLib.txt" End If |
Get details of associated Object Repositories in a Test Using AOM in UFT
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
Set qtApp = CreateObject("QuickTest.Application") Set qtRepositories = qtApp.Test.Actions("Action1").ObjectRepositories FlagObjRep=False For i = 1 To qtRepositories.Count ' Associate UFTRepository.tsr if it's not associated with the Test If qtRepositories.Item(i) = "E:\UFT_WorkSpace\SharedObjRepository\UFTRepository.tsr" Then FlagObjRep=True Exit For End If Next If FlagObjRep = False Then RepositoriesCollection.Add("E:\UFT_WorkSpace\SharedObjRepository\UFTRepository.tsr") End If |
Establishing Connection of UFT with The Quality Center (HP ALM)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
Set qtApp = CreateObject("QuickTest.Application") ' Create the Application object qtApp.Launch 'Remove the code if written inside a Test UFT qtApp.Visible = True 'Remove the code if written inside a Test UFT ' Make changes in a test on Quality Center with version control qtApp.TDConnection.Connect "QC URL", "Domain", "ProjectName", "UserName", "Pwd", False If qtApp.TDConnection.IsConnected Then ' If connection is successful Msgbox "Connected to HP ALM" Else Msgbox "Cannot connect to HP ALM" End If |
Load Terminal Emulator add-in and Emulator Options at runtime Using AOM in UFT
1 2 3 4 5 6 7 8 9 10 11 |
Set qtApp = CreateObject("QuickTest.Application") ' Create the Application object Set qtTeOptions = qtApp.Options.TE 'Return the TE options object qtTeOptions.CurrentEmulator = "Extra 6.7" qtTeOptions.Protocol = "autodetect" Set qtTeOptions = Nothing Set qtApp = Nothing |
The following setting would be done automatically using the above code.
Generate Automation Object Model (AOM) Script
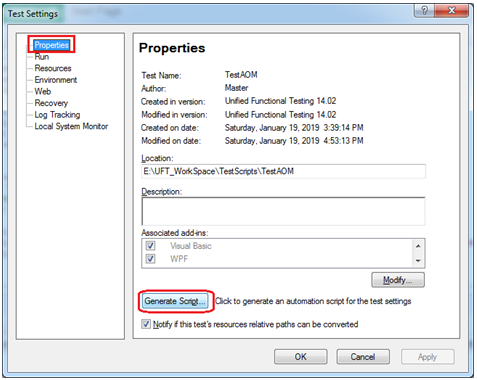
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
Dim App 'As Application Set App = CreateObject("QuickTest.Application") App.Launch App.Visible = True App.Test.Settings.Launchers("Web").Active = False App.Test.Settings.Launchers("Web").Browser = "2048" App.Test.Settings.Launchers("Web").Address = "http://newtours.demoaut.com" App.Test.Settings.Launchers("Web").CloseOnExit = True App.Test.Settings.Launchers("Web").RuntimeParameterization = 0 App.Test.Settings.Launchers("Windows Applications").Active = True App.Test.Settings.Launchers("Windows Applications").Applications.RemoveAll App.Test.Settings.Launchers("Windows Applications").RecordOnQTDescendants = True App.Test.Settings.Launchers("Windows Applications").RecordOnExplorerDescendants = False App.Test.Settings.Launchers("Windows Applications").RecordOnSpecifiedApplications = True App.Test.Settings.Run.IterationMode = "rngAll" App.Test.Settings.Run.StartIteration = 1 App.Test.Settings.Run.EndIteration = 1 App.Test.Settings.Run.ObjectSyncTimeOut = 20000 App.Test.Settings.Run.DisableSmartIdentification = False App.Test.Settings.Run.OnError = "Dialog" App.Test.Settings.Resources.DataTablePath = "" App.Test.Settings.Resources.Libraries.RemoveAll App.Test.Settings.Web.BrowserNavigationTimeout = 60000 App.Test.Settings.Web.ActiveScreenAccess.UserName = "" App.Test.Settings.Web.ActiveScreenAccess.Password = "" '''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''' ' System Local Monitoring settings '''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''' App.Test.Settings.LocalSystemMonitor.Enable = false '''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''' ' Log Tracking settings '''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''' With App.Test.Settings.LogTracking .IncludeInResults = False .Port = 18081 .IP = "127.0.0.1" .MinTriggerLevel = "ERROR" .EnableAutoConfig = False .RecoverConfigAfterRun = False .ConfigFile = "" .MinConfigLevel = "WARN" End With |
Conclusion
In this tutorial,we have seen many useful exampls of using UFT automation object model.Hope the above examples give you a fair idea of how to use AOM in UFT, please do share this post and like it.
Recommended Posts
- Component Object Model in UFT | DOM | TOM & BOM
- Mainframe Automation Using UFT One
- How to Use LoadAndRunAction in UFT
- How to Use WaitProperty | Dynamic Wait in UFT
- How To Get Tooltip Text In UFT
- How to Use Call Stack in UFT With Example
- How to Debug a Test in UFT
- How to Use XPath in UFT One To Identify Web-Based Objects
- How To Use Dictionary Object in UFT With Examples